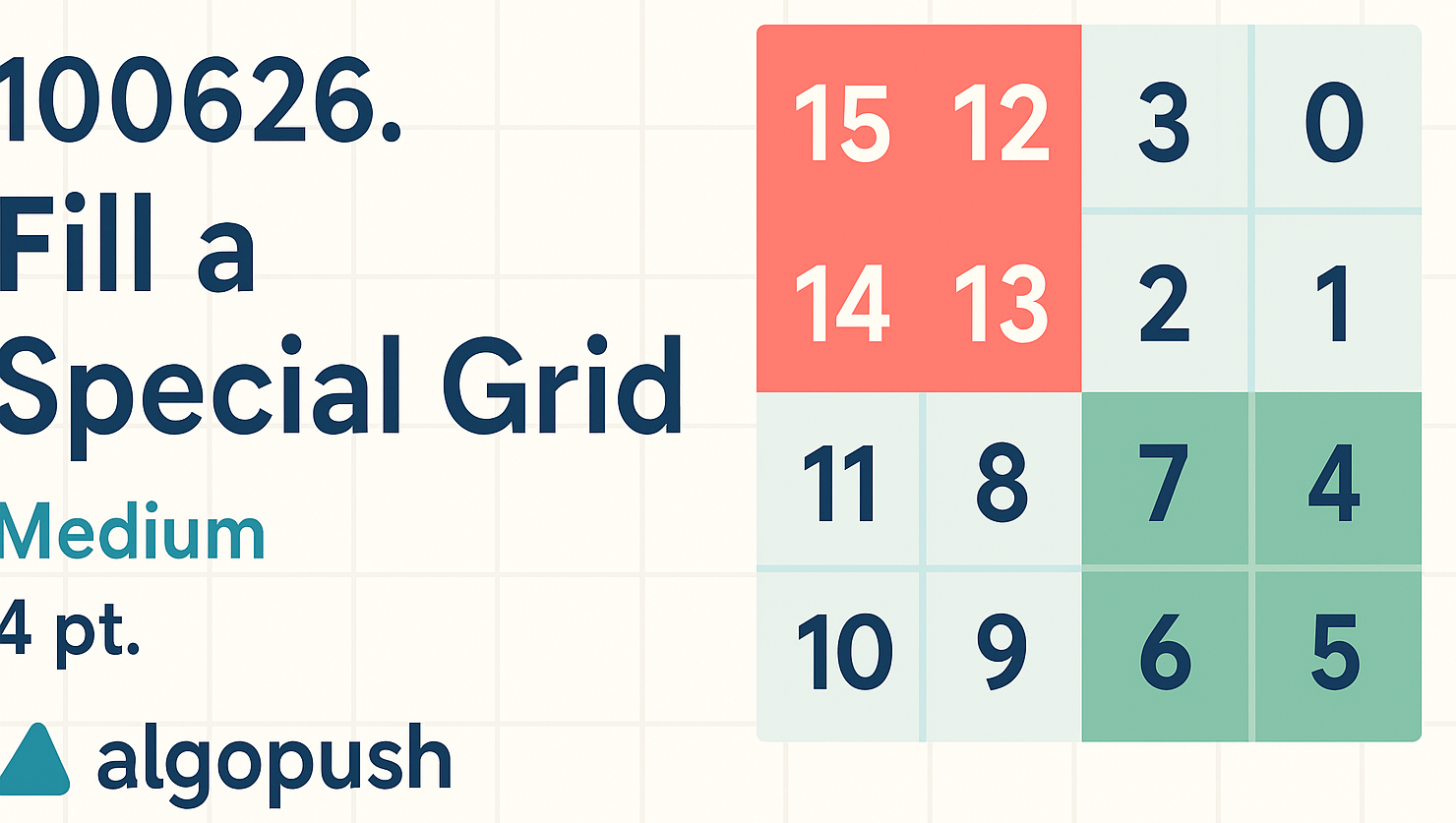
100626: Fill a Special Grid
Problem Statement
Construct a 2N x 2N grid where each quadrant follows strict ordering rules recursively. Numbers must range from 0 to 22N - 1.
Example 1
Input: N = 1
Output: [[3,0],[2,1]]
Explanation: Quadrants ordered as 0 < 1 < 2 < 3
Example 2
Input: N = 2
Output: [[15,12,3,0],[14,13,2,1],[11,8,7,4],[10,9,6,5]]
Explanation: Each quadrant maintains required ordering recursively
Approach 1: Recursive Quadrant Filling
Divide grid into quadrants and fill recursively following the ordering rules.
Algorithm Steps
- Base case: 1x1 grid contains 0
- For larger grids:
- Divide into four 2N-1 x 2N-1 quadrants
- Fill quadrants in reverse order (TR → BR → BL → TL)
- Offset values based on quadrant position
Complexity Analysis
Time Complexity | Space Complexity |
---|---|
O(4N) | O(4N) |
class Solution {
public:
vector<vector<int>> specialGrid(int N) {
int size = pow(2, N);
vector<vector<int>> grid(size, vector<int>(size));
fill(grid, 0, 0, size, 0);
return grid;
}
void fill(vector<vector<int>>& grid, int x, int y, int size, int start) {
if(size == 1) {
grid[x][y] = start;
return;
}
int half = size / 2;
int quarter = (size * size) / 4;
// Fill quadrants in TR → BR → BL → TL order
fill(grid, x, y + half, half, start); // Top-right
fill(grid, x + half, y + half, half, start + quarter); // Bottom-right
fill(grid, x + half, y, half, start + 2*quarter); // Bottom-left
fill(grid, x, y, half, start + 3*quarter); // Top-left
}
};
class Solution {
public int[][] specialGrid(int N) {
int size = (int) Math.pow(2, N);
int[][] grid = new int[size][size];
fill(grid, 0, 0, size, 0);
return grid;
}
private void fill(int[][] grid, int x, int y, int size, int start) {
if(size == 1) {
grid[x][y] = start;
return;
}
int half = size / 2;
int quarter = (size * size) / 4;
fill(grid, x, y + half, half, start); // Top-right
fill(grid, x + half, y + half, half, start + quarter); // Bottom-right
fill(grid, x + half, y, half, start + 2*quarter); // Bottom-left
fill(grid, x, y, half, start + 3*quarter); // Top-left
}
}
class Solution:
def specialGrid(self, N: int) -> List[List[int]]:
size = 2 ** N
grid = [[0] * size for _ in range(size)]
self.fill(grid, 0, 0, size, 0)
return grid
def fill(self, grid, x, y, size, start):
if size == 1:
grid[x][y] = start
return
half = size // 2
quarter = (size * size) // 4
self.fill(grid, x, y + half, half, start) # Top-right
self.fill(grid, x + half, y + half, half, start + quarter) # Bottom-right
self.fill(grid, x + half, y, half, start + 2*quarter) # Bottom-left
self.fill(grid, x, y, half, start + 3*quarter) # Top-left
Approach 2: Mathematical Pattern Construction
Use bit manipulation and coordinate patterns to calculate values directly.
Algorithm Steps
- For each cell (i,j):
- Determine quadrant level
- Calculate value based on quadrant position
- Use bitwise operations to mirror coordinates
Complexity Analysis
Time Complexity | Space Complexity |
---|---|
O(4N) | O(4N) |
class Solution {
public:
vector<vector<int>> specialGrid(int N) {
int size = pow(2, N);
vector<vector<int>> grid(size, vector<int>(size));
for(int i = 0; i < size; ++i) {
for(int j = 0; j < size; ++j) {
int val = 0;
for(int k = N-1; k >= 0; --k) {
int mask = 1 << k;
int x = (i & mask) ? 1 : 0;
int y = (j & mask) ? 1 : 0;
val = (val << 2) | ((3 - 2*x - y) & 3);
}
grid[i][j] = val;
}
}
return grid;
}
};
class Solution {
public int[][] specialGrid(int N) {
int size = (int) Math.pow(2, N);
int[][] grid = new int[size][size];
for(int i = 0; i < size; ++i) {
for(int j = 0; j < size; ++j) {
int val = 0;
for(int k = N-1; k >= 0; --k) {
int mask = 1 << k;
int x = (i & mask) != 0 ? 1 : 0;
int y = (j & mask) != 0 ? 1 : 0;
val = (val << 2) | ((3 - 2*x - y) & 3);
}
grid[i][j] = val;
}
}
return grid;
}
}
class Solution:
def specialGrid(self, N: int) -> List[List[int]]:
size = 2 ** N
grid = [[0] * size for _ in range(size)]
for i in range(size):
for j in range(size):
val = 0
for k in range(N-1, -1, -1):
mask = 1 << k
x = (i & mask) != 0
y = (j & mask) != 0
val = (val << 2) | ((3 - 2*x - y) & 3)
grid[i][j] = val
return grid
Edge Cases and Special Considerations
Key edge cases to consider when implementing your solution:
1. Base Case (N=0)
Input: 0 → Output: [[0]]
Explanation: Single cell grid with value 0
2. N=1 Verification
Input: 1 → Output: [[3,0],[2,1]]
Explanation: Verify quadrant ordering TR=0, BR=1, BL=2, TL=3
3. Large N Values
Input: 3 → Output: 8x8 grid
Explanation: Ensure recursive pattern holds for deep nesting
Frequently Asked Questions
1. Why does the recursive approach work?
The recursive approach maintains the quadrant ordering by assigning value ranges that ensure each quadrant's maximum is less than the next quadrant's minimum.
2. How does the mathematical solution work?
It encodes quadrant positions at each level using bit patterns, calculating the value through bitwise operations that mirror the recursive structure.
3. Can we optimize further?
Both approaches are optimal for their paradigms. The mathematical solution avoids recursion stack overhead but may be harder to understand.
4. How to handle very large N?
Both approaches work up to N=15 (32768x32768 grid) within memory limits. For N>15, consider iterative approaches with memory optimization.
5. Why reverse order in recursion?
Filling TR first ensures smaller values are placed first, followed by larger values in subsequent quadrants to maintain the required ordering.