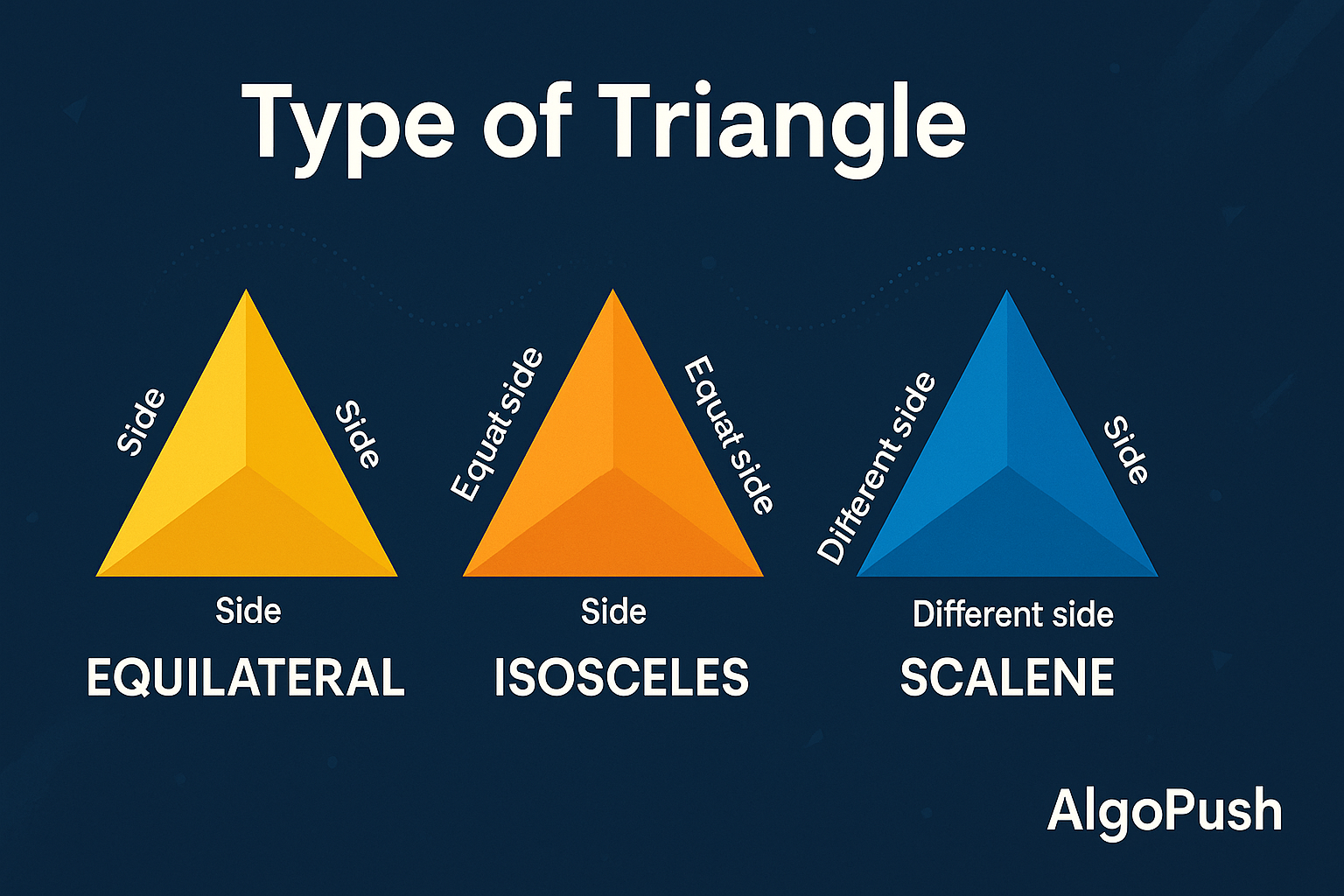
3024: Type of Triangle
Problem Statement
Given three positive integers representing triangle sides, classify the triangle type (equilateral, isosceles, scalene) or return "none" if invalid.
Example 1
Input: [3,3,3] → Output: "equilateral"
Example 2
Input: [3,4,5] → Output: "scalene"
Approach 1: Direct Comparison
Check all three triangle inequalities directly and compare side lengths.
Algorithm Steps
- Check validity using a + b > c for all combinations
- Check for equilateral (all sides equal)
- Check for scalene (all sides different)
- Default to isosceles if exactly two sides match
Complexity Analysis
Time | Space |
---|---|
O(1) | O(1) |
class Solution {
public:
string triangleType(vector<int>& nums) {
int a = nums[0], b = nums[1], c = nums[2];
if(a + b <= c || b + c <= a || c + a <= b) return "none";
if(a == b && b == c) return "equilateral";
if(a != b && b != c && a != c) return "scalene";
return "isosceles";
}
};
class Solution {
public String triangleType(int[] nums) {
int a = nums[0], b = nums[1], c = nums[2];
if(a + b <= c || b + c <= a || c + a <= b) return "none";
if(a == b && b == c) return "equilateral";
if(a != b && b != c && a != c) return "scalene";
return "isosceles";
}
}
class Solution:
def triangleType(self, nums: List[int]) -> str:
a, b, c = nums
if a + b <= c or b + c <= a or c + a <= b:
return "none"
if a == b == c:
return "equilateral"
if a != b and b != c and a != c:
return "scalene"
return "isosceles"
Approach 2: Sorting Optimization
Sort sides first to simplify validity check to a single condition.
Algorithm Steps
- Sort the array in ascending order
- Check if smallest two sides sum > largest side
- Use set to count unique values for classification
class Solution {
public:
string triangleType(vector<int>& nums) {
sort(nums.begin(), nums.end());
if(nums[0] + nums[1] <= nums[2])
return "none";
if(nums[0] == nums[2])
return "equilateral";
if(nums[0] == nums[1] || nums[1] == nums[2])
return "isosceles";
return "scalene";
}
};
class Solution {
public String triangleType(int[] nums) {
Arrays.sort(nums);
if(nums[0] + nums[1] <= nums[2])
return "none";
if(nums[0] == nums[2])
return "equilateral";
if(nums[0] == nums[1] || nums[1] == nums[2])
return "isosceles";
return "scalene";
}
}
class Solution:
def triangleType(self, nums: List[int]) -> str:
nums.sort()
if nums[0] + nums[1] <= nums[2]:
return "none"
if nums[0] == nums[2]:
return "equilateral"
if nums[0] == nums[1] or nums[1] == nums[2]:
return "isosceles"
return "scalene"
Approach 3: Set-Based Detection
Use Set to track unique values for efficient type classification.
class Solution {
public:
string triangleType(vector<int>& nums) {
unordered_set<int> s(nums.begin(), nums.end());
if(nums[0] + nums[1] <= nums[2] ||
nums[1] + nums[2] <= nums[0] ||
nums[2] + nums[0] <= nums[1])
return "none";
if(s.size() == 1) return "equilateral";
if(s.size() == 2) return "isosceles";
return "scalene";
}
};
class Solution {
public String triangleType(int[] nums) {
Set<Integer> set = new HashSet<>();
for(int num : nums) set.add(num);
if(nums[0] + nums[1] <= nums[2] ||
nums[1] + nums[2] <= nums[0] ||
nums[2] + nums[0] <= nums[1])
return "none";
if(set.size() == 1) return "equilateral";
if(set.size() == 2) return "isosceles";
return "scalene";
}
}
class Solution:
def triangleType(self, nums: List[int]) -> str:
unique = len(set(nums))
a, b, c = nums
if a + b <= c or b + c <= a or c + a <= b:
return "none"
if unique == 1: return "equilateral"
if unique == 2: return "isosceles"
return "scalene"
Approach Comparison
Approach | Time | Space | Readability | Check Count |
---|---|---|---|---|
Direct Comparison | O(1) | O(1) | Good | 3 validity checks |
Sorting | O(1)* | O(1) | Best | 1 validity check |
Set-Based | O(1) | O(1) | Moderate | 3 validity checks |
* Sorting 3 elements is effectively O(1)
Edge Cases
1. Invalid Triangle
Input: [1,2,3] → Output: "none"
2. Two Equal Sides (Isosceles)
Input: [3,3,4] → Output: "isosceles"
3. All Sides Equal
Input: [5,5,5] → Output: "equilateral"
4. Zero Length (Invalid by Constraints)
Input: [0,1,1] → Constraints prevent this
Frequently Asked Questions
Why check a + b > c instead of ≥?
Triangle inequality theorem requires the sum of any two sides to be strictly greater than the third side for a valid triangle.
Does the order of sides matter?
No, triangle properties are order-agnostic. All approaches handle any order of input sides.
How does sorting help in validation?
After sorting, we only need to check if the sum of the two smaller sides is greater than the largest side.
Why use a Set in Approach 3?
A Set automatically tracks unique values, making it efficient to determine the number of distinct sides.
Can we have multiple correct answers?
No, each valid triangle has exactly one correct classification based on side lengths.
How to handle floating-point numbers?
While the problem specifies integers, the same logic works for floating-point numbers using appropriate comparisons.
What's the maximum input size?
Fixed input size of 3 elements (nums.length == 3) as per problem constraints.
Why O(1) space complexity?
All approaches use constant extra space regardless of input size (fixed to 3 elements).
Can we use bit manipulation?
Possible but would complicate the solution without providing any performance benefits.
How to extend for polygons?
This solution is specific to triangles. Polygons require different validation (sum of (n-1) sides > longest side).
Why prefer sorting approach?
Reduces validity checks from 3 to 1, improves readability, and handles side order automatically.
What about negative numbers?
Problem constraints specify 1 ≤ nums[i] ≤ 100, making negative inputs impossible.