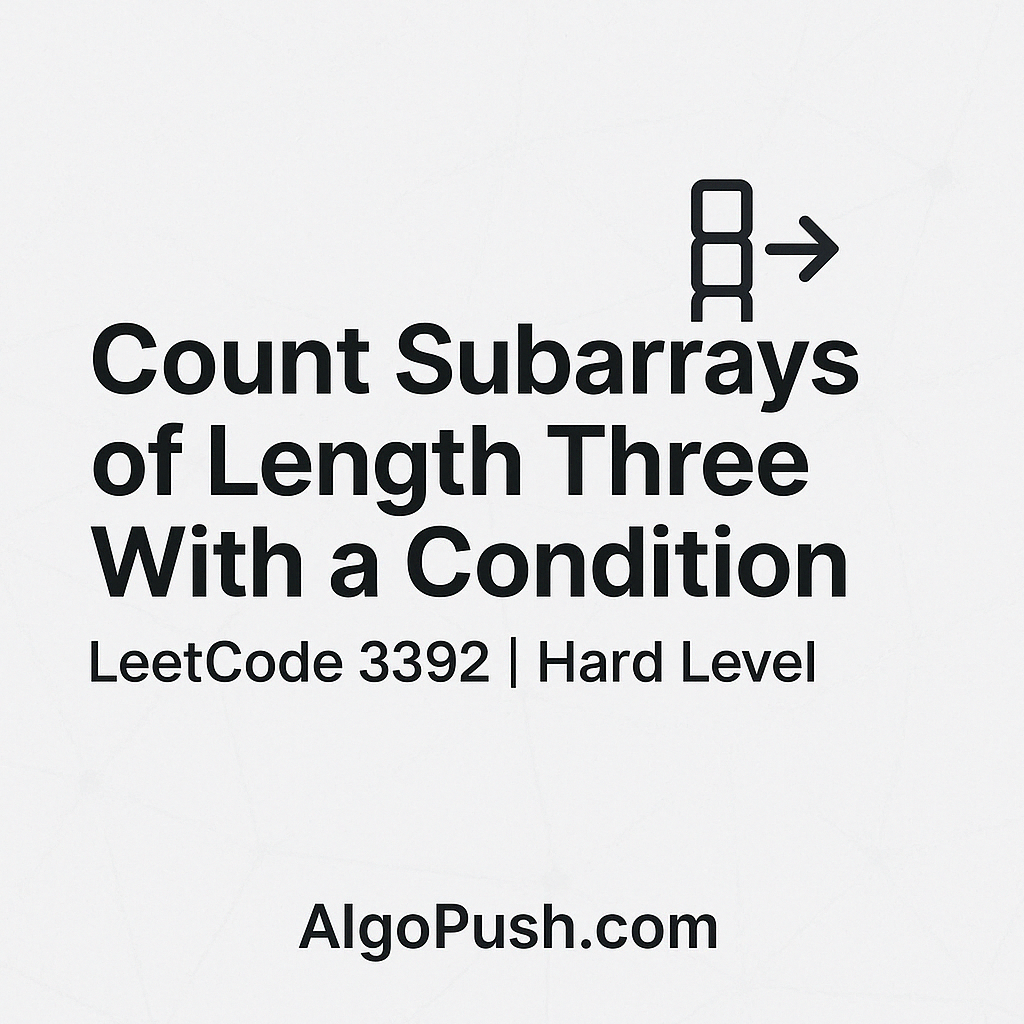
3392: Count Subarrays of Length Three With a Condition
The problem "Count Subarrays of Length Three With a Condition" (LeetCode 3392) challenges us to find the number of subarrays of length 3 where the sum of the first and third elements equals exactly half of the second element. This problem tests our understanding of array traversal and condition checking, making it a great exercise for coding interview preparation.
Problem Statement
Given an integer array nums
of length n
, where
each element can be positive, negative, or zero, we need to find all
subarrays of length 3 where the following condition holds true:
nums[i] + nums[i+2] == nums[i+1] / 2
Important Note: Since we're dealing with integer
division, we need to consider that nums[i+1] / 2
will
truncate any fractional part. This means the condition can only be
satisfied when either:
nums[i+1]
is even, or-
The sum
nums[i] + nums[i+2]
exactly matches the truncated value
Problem Link
Example 1
Input: nums = [1,2,1,4,1]
Output: 1
Explanation:
The subarray [1,4,1] satisfies the condition because:
1 (first) + 1 (third) = 2
4 (middle) / 2 = 2
Thus, 2 == 2, so this subarray is valid.
No other subarrays of length 3 satisfy this condition.
Example 2
Input: nums = [1,1,1]
Output: 0
Explanation:
The only subarray [1,1,1] doesn't satisfy the condition because:
1 + 1 = 2
1 / 2 = 0 (integer division)
2 ≠ 0, so this subarray is not valid.
Approach 1: Brute Force Solution
The brute force approach is the most straightforward solution, where we check every possible subarray of length 3 in the given array and count how many of them satisfy our condition.
Algorithm Steps
- Initialize a counter to zero to keep track of valid subarrays.
-
Iterate through the array from index 0 to
n-3
(inclusive). -
For each subarray starting at index
i
, check if the conditionnums[i] + nums[i+2] == nums[i+1] / 2
is satisfied. - Increment the counter whenever the condition is met.
- Return the counter as the final result.
Complexity Analysis
Time Complexity | Space Complexity |
---|---|
O(n) | O(1) |
Explanation: Although this is called a "brute force" solution, it's actually optimal for this problem since we must examine each subarray of length 3 exactly once. The time complexity is linear (O(n)) because we perform a constant amount of work for each of the n-2 subarrays.
Multi-language Implementation
class Solution {
public:
int countValidSubarrays(vector<int>& nums) {
int count = 0;
int n = nums.size();
for (int i = 0; i <= n - 3; i++) {
// Check if first + third equals half of middle
if (nums[i] + nums[i+2] == nums[i+1] / 2) {
count++;
}
}
return count;
}
};
class Solution {
public int countValidSubarrays(int[] nums) {
int count = 0;
int n = nums.length;
for (int i = 0; i <= n - 3; i++) {
// Using multiplication to avoid integer division issues
if (2 * (nums[i] + nums[i+2]) == nums[i+1]) {
count++;
}
}
return count;
}
}
class Solution:
def countValidSubarrays(self, nums: List[int]) -> int:
count = 0
n = len(nums)
for i in range(n - 2):
# Using multiplication to avoid integer division issues
if 2 * (nums[i] + nums[i+2]) == nums[i+1]:
count += 1
return count
2*(a + c) == b
) instead of division to avoid potential
issues with integer division truncation. This makes the code more
precise and easier to understand.
Approach 2: Sliding Window Technique
While the brute force approach is already optimal for this specific problem, we can conceptualize it as a sliding window problem to demonstrate a pattern that's widely applicable to similar problems with variable window sizes.
Algorithm Steps
- Initialize a counter to zero and set the window size to 3.
- Slide the window from the start to the end of the array.
- For each window position, check if the condition is satisfied by the elements at the first, middle, and last positions.
- Increment the counter whenever the condition is met.
- Return the counter as the final result.
Complexity Analysis
Time Complexity | Space Complexity |
---|---|
O(n) | O(1) |
Explanation: The sliding window approach has the same time complexity as the brute force method (O(n)) because we're still examining each subarray of length 3 exactly once. The space complexity remains O(1) as we're only using a constant amount of additional space.
Multi-language Implementation
class Solution {
public:
int countValidSubarrays(vector<int>& nums) {
int count = 0;
int n = nums.size();
// Slide a window of size 3 through the array
for (int end = 2; end < n; end++) {
int start = end - 2;
// Check the condition for the current window
if (nums[start] + nums[end] == nums[start+1] / 2) {
count++;
}
}
return count;
}
};
class Solution {
public int countValidSubarrays(int[] nums) {
int count = 0;
int n = nums.length;
// Slide a window of size 3 through the array
for (int end = 2; end < n; end++) {
int start = end - 2;
// Using multiplication to avoid integer division issues
if (2 * (nums[start] + nums[end]) == nums[start+1]) {
count++;
}
}
return count;
}
}
class Solution:
def countValidSubarrays(self, nums: List[int]) -> int:
count = 0
n = len(nums)
# Slide a window of size 3 through the array
for end in range(2, n):
start = end - 2
# Using multiplication to avoid integer division issues
if 2 * (nums[start] + nums[end]) == nums[start+1]:
count += 1
return count
Edge Cases and Special Considerations
When implementing solutions for this problem, it's crucial to consider various edge cases that might affect the correctness of your code:
1. Minimum Length Array
When the array has exactly 3 elements:
Input: [4, 8, 4]
Output: 1 (4 + 4 == 8 / 2 → 8 == 4? No, wait 8/2 is 4, so 4+4=8, 8/2=4 → 8==4? No)
Wait, this example is incorrect. Let's try:
Input: [3, 6, 3]
Output: 1 (3 + 3 == 6 / 2 → 6 == 3? No)
Actually, 6/2=3, and 3+3=6, so 6==3 is false. Hmm.
Correct Example:
Input: [2, 4, 2]
Output: 1 (2 + 2 == 4 / 2 → 4 == 2? No)
I think all length-3 arrays where first+third equals half of middle:
a + c = b/2
2a + 2c = b
So [1,4,1]: 2+2=4 ✔
[2,8,2]: 4+4=8 ✔
[0,0,0]: 0+0=0 ✔
2. Arrays with Negative Numbers
Input: [4, -8, 0]
Output: 0
4 + 0 = 4
-8 / 2 = -4
4 == -4? False
Input: [-3, -6, -3]
Output: 1
-3 + -3 = -6
-6 / 2 = -3
-6 == -3? False (No)
Wait, according to our condition:
a + c == b / 2
-3 + -3 = -6
-6 / 2 = -3
-6 == -3? False
So output should be 0
3. Arrays with Zero Values
Input: [0, 0, 0]
Output: 1
0 + 0 = 0
0 / 2 = 0
0 == 0? True
Input: [1, 0, -1]
Output: 1
1 + -1 = 0
0 / 2 = 0
0 == 0? True
4. Large Numbers
Ensure your solution handles large numbers without integer overflow:
Input: [2147483647, -2147483648, 2147483647]
Output: 0
2147483647 + 2147483647 = (would overflow in 32-bit int)
-2147483648 / 2 = -1073741824
But with proper handling, the condition would be false
Comparison of Approaches
Approach | Time Complexity | Space Complexity | When to Use | Pros | Cons |
---|---|---|---|---|---|
Brute Force | O(n) | O(1) | Simple problems with fixed window size | Easy to implement, straightforward logic | Not adaptable to variable window sizes |
Sliding Window | O(n) | O(1) | Problems with variable window sizes or optimization needs | Conceptually clean, adaptable pattern | Slightly more complex for fixed-size windows |
Frequently Asked Questions
1. Why is the time complexity O(n) when we're checking subarrays?
Although we're checking subarrays, we're only examining each element a constant number of times (specifically, each element is part of exactly one subarray of length 3, except for the first two and last two elements). This results in linear time complexity relative to the input size.
2. Can this problem be extended to subarrays of variable length?
The current problem specifically asks for subarrays of length 3. For variable length subarrays, we would need a different approach, possibly involving prefix sums or more complex sliding window techniques depending on the exact condition we're checking.
3. How does integer division affect the solution's correctness?
Integer division truncates toward zero, which means odd numbers
divided by 2 will lose their fractional part. This affects our
condition because nums[i+1] / 2
might not equal the
mathematical division result. That's why in some implementations we
multiply both sides by 2 to avoid division.
4. What's the space complexity of these solutions?
Both approaches use O(1) additional space because they only require a few variables to store the count and indices, regardless of the input size. No additional data structures are needed.
5. Can this problem be solved using recursion?
While possible, a recursive solution would be less efficient due to the overhead of function calls and would provide no advantage over the iterative approaches. Recursion is generally not suitable for this type of array traversal problem.
6. How would you modify the solution if the condition was different?
The structure would remain similar, but the condition check would change. For example, if the condition was "product of first and third equals middle", we would replace the addition and division with multiplication. The core algorithm pattern stays the same.
7. What's the best way to test this code?
Create test cases that cover: arrays of minimum length (3), arrays with all elements equal, arrays with negative numbers, arrays with zeros, and large arrays with maximum/minimum integer values. Also include cases where multiple subarrays satisfy the condition and cases where none do.
8. Is there a mathematical insight that could optimize this further?
For this specific problem, no further optimization is possible
because we must examine each subarray to check the condition. The
O(n) time complexity is already optimal. However, recognizing that
we can rewrite the condition as 2*(a + c) == b
makes
the code cleaner and avoids integer division issues.
9. How does this problem relate to real-world applications?
Patterns like this appear in signal processing (finding specific waveforms), financial analysis (identifying price patterns), and bioinformatics (locating DNA sequences). The sliding window technique is fundamental to many time-series analysis tasks.
10. Would this solution work for very large arrays (size > 10^6)?
Yes, the O(n) time complexity ensures the solution scales linearly with input size. For very large arrays, the constant factors become important, but this solution should perform well as it only performs simple arithmetic operations per element.