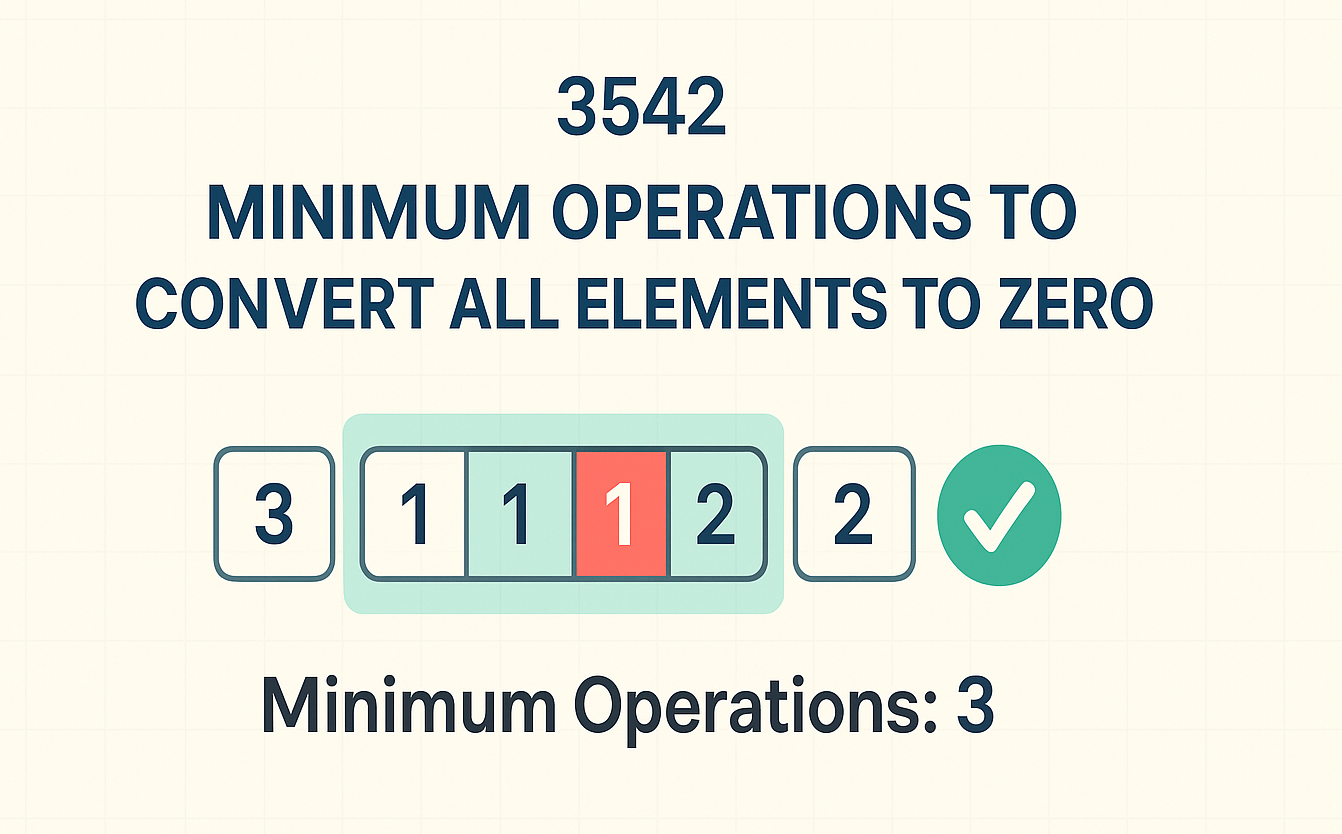
3542: Minimum Operations to Convert All Elements to Zero
Problem Statement
Given an array of non-negative integers, determine the minimum number of operations needed to make all elements zero. Each operation lets you select a subarray and set all occurrences of its minimum value to zero.
Example 1
Input: nums = [0,2]
Output: 1
Explanation: Target subarray [1,1] containing 2
Example 2
Input: nums = [3,1,2,1]
Output: 3
Explanation: Requires three targeted operations
Approach 1: Greedy Group Counting (Optimal)
Process elements in sorted order, counting contiguous groups for each value.
Algorithm Steps
- Sort unique values in ascending order
- For each value v:
- Count contiguous groups of v separated by higher values/zeros
- Add group count to total operations
Complexity Analysis
Time Complexity | Space Complexity |
---|---|
O(n log n) | O(n) |
class Solution {
public:
int minOperations(vector<int>& nums) {
set<int> unique(nums.begin(), nums.end());
vector<int> sorted(unique.begin(), unique.end());
int total = 0;
for(int v : sorted) {
if(v == 0) continue;
int count = 0;
bool inGroup = false;
for(int num : nums) {
if(num >= v) {
if(num == v && !inGroup) {
count++;
inGroup = true;
}
if(num != v) inGroup = false;
} else {
inGroup = false;
}
}
total += count;
}
return total;
}
};
class Solution {
public int minOperations(int[] nums) {
Set<Integer> unique = new HashSet<>();
for(int num : nums) unique.add(num);
List<Integer> sorted = new ArrayList<>(unique);
Collections.sort(sorted);
int total = 0;
for(int v : sorted) {
if(v == 0) continue;
int count = 0;
boolean inGroup = false;
for(int num : nums) {
if(num >= v) {
if(num == v && !inGroup) {
count++;
inGroup = true;
}
if(num != v) inGroup = false;
} else {
inGroup = false;
}
}
total += count;
}
return total;
}
}
class Solution:
def minOperations(self, nums: List[int]) -> int:
unique = sorted(set(nums))
total = 0
for v in unique:
if v == 0:
continue
count = 0
in_group = False
for num in nums:
if num >= v:
if num == v and not in_group:
count += 1
in_group = True
if num != v:
in_group = False
else:
in_group = False
total += count
return total
Approach Comparison
Approach | Time | Space | Use Case |
---|---|---|---|
Greedy Group Counting | O(n log n) | O(n) | General case, optimal solution |
Frequency Map | O(n²) | O(n) | Theoretical alternative |
Edge Case Analysis
1. All Elements Zero
Input: [0,0,0] → Output: 0
2. Strictly Increasing
Input: [1,2,3,4] → Output: 4
3. Alternating Values
Input: [2,1,2,1] → Output: 2
Frequently Asked Questions
1. Why process values in sorted order?
Smaller values can be eliminated first, creating segments that reduce operations needed for larger values.
2. How does group counting work?
Contiguous blocks of the same value separated by higher values/zeros each require one operation.
3. Why O(n log n) complexity?
Due to sorting unique values. Linear scans afterward maintain efficiency.
4. Can we optimize further?
This is already optimal for constraints. Further optimization would require different mathematical modeling.
5. How handle duplicate values?
Duplicates in the same contiguous group are handled in a single operation.
6. Why use set for unique values?
Ensures we process each value exactly once, avoiding redundant calculations.
7. What if all elements are same?
Requires exactly 1 operation regardless of array size.
8. How verify correctness?
Check that each operation reduces the maximum value until all elements are zero.
9. Why track 'inGroup' state?
Prevents overcounting by tracking contiguous value sequences efficiently.
10. Real-world applications?
Resource deallocation, memory management, and optimization problems in distributed systems.